Inheritance
Association
Dependency
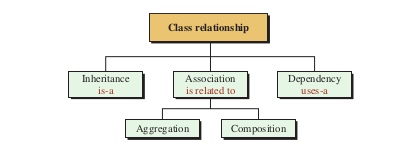
Inheritance
Association
Dependency
The 2nd principle of Object Oriented Programming
We use Unified Modeling Language (UML) to shows the relationship between classes & objects graphically
Base class or superclass - the general class
Derived class or subclass - a specific class
A derived class extends its base class;
UML diagram for inheritance
Types of inheritance in C++;
Use (:) after a base class (e.g. B-base & D-derived class)
Types of inheritance
A private member in the base class becomes an inaccessible (hidden) member in the derived class
A public member in the base class becomes a public member in the derived class
Public inheritance is the most common. e.g.
Protected inheritance is rare and virtually never used
Private inheritance does not define a type inheritance; it defines an implementation inheritance
Public members of the base class become private members in the derived class
This property allows inherited implementation (code reuse)
Forms of inheritance
Bitbucket: Multiple inheritance
Bitbucket: Multilevel inheritance
Bitbucket: Hierarchical inheritance
Home: Hierarchical inheritance
Not all relationships between classes can be defined as inheritance
it models the has-a relationship
An association between two classes shows a relationship. For example, we can define a class named Person and another class named Address
A person lives in an address and the address is occupied by a person. The Address class is not inherited from the Person class; neither the other way
1. Aggregation
Example
2. Composition
Dependency is a weaker relationship than inheritance or association
Dependency models the uses relationship
For Class A & B, dependency happens when
Queries about this Lesson, please send them to:
*References*
- C++ Programming - An Object-Oriented Approach, 2019
Behrouz, Richard et.al
- Accelerated C++ - Practical Programming by Example, 2000
Andrew, Barbara et.al
Courtesy of …